TypeScript Experts
Hire the best TypeScript & JavaScript engineers remotely
What is TypeScript?
TypeScript is a programming language co-engineered by Anders Hejlsberg (designer of C#, Turbo Pascal, and Delphi) and maintained by Microsoft. The first specification appeared eight years ago.
It supercharges JavaScript with an optional typing system making it an excellent language for enterprise-level and small-sized applications.
Because TypeScript is a superset of JavaScript, all JavaScript code is a valid TypeScript. That feature makes TypeScript very accessible and easy to adopt in existing projects. And since JavaScript is one of the most widely used languages globally, it comes with no surprise that TypeScript is now also becoming (2021) one of the most popular languages.
Who uses TypeScript?
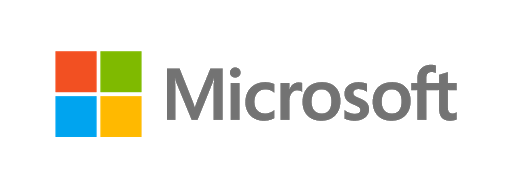
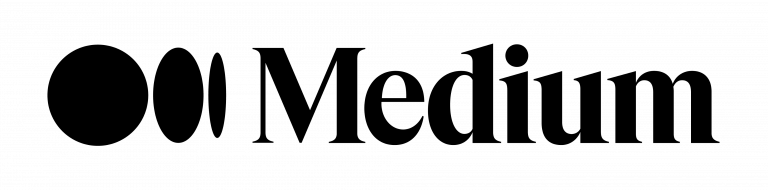
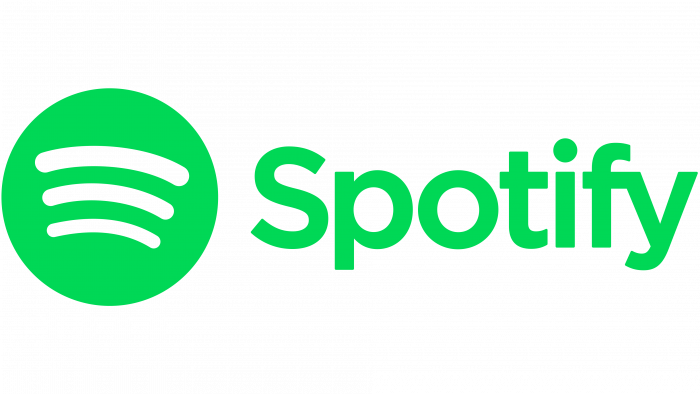
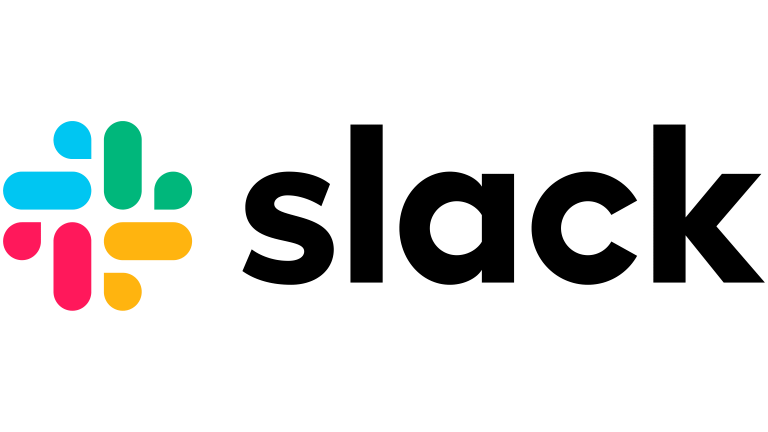
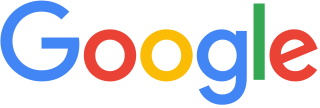
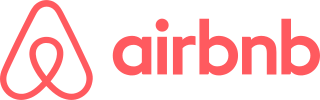
How does TypeScript work?
TypeScript is a statically typed language. It means that the compiler will check the types for you. After compiling (or, actually, transpiling), the code becomes standard JavaScript with no type annotations and runs on every JavaScript-enabled environment:
- web browsers,
- server (node.js / deno),
- mobile phones (WebView, React Native)
- desktop (Electron).
Importantly, type-checking is optional. Even though the transpiler will complain that there is a mismatch in your types, it will correctly transpile it to JavaScript, and you will be able to run it, which means that onboarding to TypeScript is seamless.
The language is often described as a superset of JavaScript. The consequence of that is that every JavaScript file is a correct TypeScript file. It is also a foundation for a significant implication: TypeScript can be progressively introduced in existing projects!
Interested in diving deeper into how to start? Here are official Get Started guides for programmers of different languages
Why should I use TypeScript in my project?
TypeScript code is scalable
Scaling an application is hardly possible without support from a programming language. Similarly, a programming language may become a bottleneck when you want to scale your team. TypeScript’s typing support will help with growing and maintaining your codebase. Since TypeScript code is usually more readable than plain JavaScript, it’s easier to onboard new team members, thus scaling your team.
With its native support of interfaces, classes, generics, and other object-oriented features, it gives you the robustness of enterprise languages such as Java or C# while retaining JavaScript’s flexibility.
The extent to which you want to use the typing system is up to you. However, TypeScript enables a design-oriented development which generally leads to a cleaner, well-thought-through code, decreases the risk of spaghetti-code, and eventually saves you time.
TypeScript can prevent bugs and decrease debugging time
In JavaScript, it’s easy to make simple mistakes that will remain well hidden until they threw an error in the runtime! Even though programmers cover their code with tests, it’s not uncommon to see bugs leaking into production. Many of such bugs could be spotted in advance if the code used static types. Not to mention that debugging type-related issues in a large-scale JavaScript application can sometimes take many hours.
Because TypeScript has mechanisms that disallow, for instance, assigning a text value to a numeric value, it will spot many errors while writing the code. Below is a simple example that illustrates what would happen if you tried to calculate a product’s discounted price incorrectly. The first two examples show JavaScript code (a buggy one and a potential fix), and the last one shows how eight extra characters in TypeScript can prevent this simple mistake.
// code.js - buggy
const discountRate = 0.2;
function calculateDiscounted(price) {
return price * (1-discountRate);
}
// The below will output NaN! Ooops - definitely not the price your customer wanted to see!
console.log(calculateDiscounted('40$'));
// code.js - fixed
const discountRate = 0.2;
function calculateDiscounted(price) {
// Extra code is required
if (typeof price !== 'number') {
throw new Error('Only numbers are supported.');
}
return price * (1-discountRate);
}
console.log(calculateDiscounted('$40'));
// code.ts - TypeScript code
const discountRate = 0.2;
// Explicitly require a "number" type
function calculateDiscounted(price: number) {
return price * (1-discountRate);
}
// TypeScript will warn you that calculateDiscounted expects a number
console.log(calculateDiscounted('$40'));
It's easy to migrate JavaScript to TypeScript
Because all of your JavaScript code is already a TypeScript code, you have already made the first step! When you eventually decide to move to TypeScript, you have several options to gradually introduce it to your project and with a limited “blast radius”. Some of your options are:
- Single file: add “//@ts-check” to a particular file – narrows the scope to that file.
- JSDocs: add types with JSDocs to existing JavaScript files.
- Type declaration files: you can start from adding .d.ts files and import them with “//@typedef {import}”.
- Flags: keep default flags, such as “–noImplicitAny” set to false. That will allow your code to follow less strict rules.
To check your TypeScript code, you will need to install a transpiler. However, if you use an editor such as VSCode, you get a build-in typescript service that enables IntelliSense out of the box (heads up, the build-in service is independent of the installed one).
Note: you can control the adoption of TypeScript in your team to the level of a file as well as specific strictness! It means that the migration is pretty much effortless!
For example, you may want to keep loose rules for some time to create a proof of concept quickly. Most likely, you won’t want to spend time polishing types at that point.
The option to skip typing system is a great advantage over strongly typed languages like Java or C# to create quick prototypes. As soon as you find the idea to be valuable, you don’t need to migrate to a different language. You can introduce stronger typing into TypeScript code when you need to increase maintainability and not be forced by the language to do it when it’s just a waste of time.
The above makes it a perfect language for startups and enterprise-grade projects.
Want to migrate JavaScript to TypeScript? Check out the official migration guide!
Share your code between front-end and back-end
Have you ever had to write two implementations of something very similar only because you used two different languages? If you use JavaScript in the frontend and (say) Python in the backend, chances are you had to duplicate some work!
For example, input data validation, algorithms, logic, supporting libraries, GraphQL queries, database access – all have areas that can be shared. What happens when you need to modify or fix them? You will need to switch back-and-forth between projects, synchronize deployments, and… have a team that can handle both languages. Think about how it increases your implementations costs, maintenance, bugs amount, and finally, client frustration.
With TypeScript, you can easily share common parts of your code in libraries, npm packages, or even directly in mono-repos. Your features and fixes are implemented once, and almost immediately available in different applications.
Thanks to the typing system, all incompatibilities of your changes are going to be spotted quickly. Besides, since you’d be are using the same language and the same runtime virtual machine, your code works the same each time!
Part of the biggest programming community in the world - JavaScript!
Undoubtedly, compared to other eco-systems, JavaScript has the largest community in the world! For example, JavaScript is the top tag on StackOverflow and the 2020 tech survey conducted by the same company provides more proofs. According to that survey, JavaScript is the most widely used language in the world.
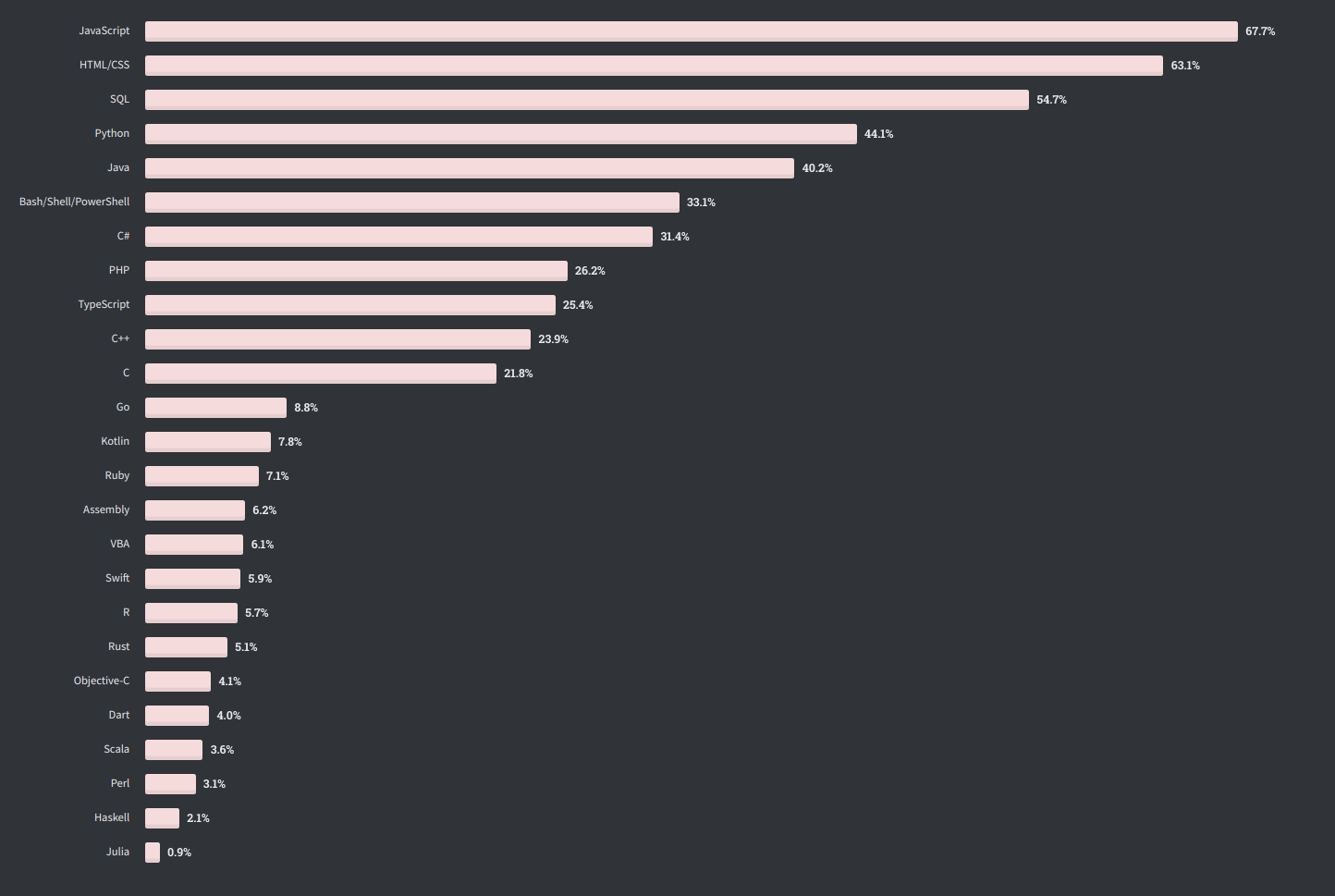
NPM – the JavaScript module registry – has the most packages among all popular languages (see chart on the right). More tools, more choices, higher productivity!
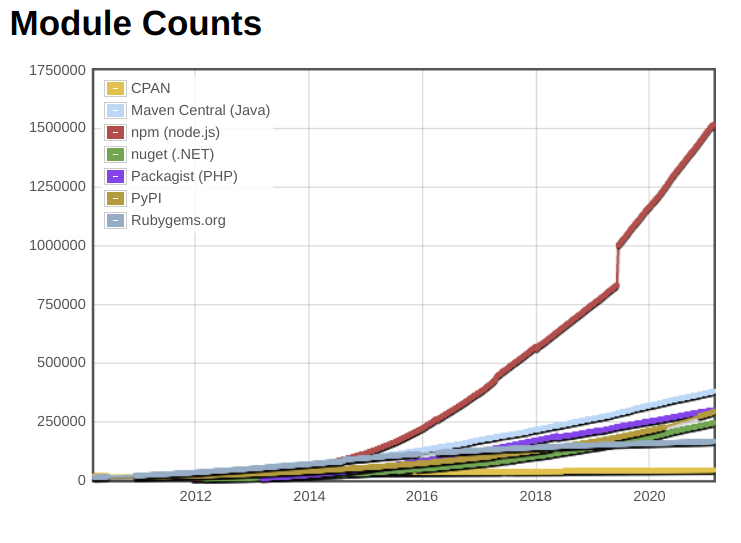
Because JavaScript is so popular, most language-related problems are solved and can be found on programming forums, social media groups, and blogs. Not only it’s easy to google an ad-hoc problem but there are also hundreds of courses, blogs, tutorials, and other educational resources available for free that help to learn the language.
Because of its nature, all of the above applies to TypeScript as well. Many libraries, including the most important ones, are shipped with type-definition files describing their interface. If a developer didn’t ship the types it is likely someone else voluntarily pushed them to DefinitelyTyped project. Actually, it is hard to find even fairly popular modules not having the pre-made types.
TypeScript - one of the most loved programming language
TypeScript has been gaining popularity fast in recent years, and surveys from 2020 show that it is now becoming one of the most loved, most popular, most widely used languages.
Our observations suggest that it will continue to widespread in the market over the next years. Several facts back this opinion up.
- It originates from JavaScript, which is the most popular language and is relatively easy to learn and use.
- It is maintained by Microsoft and popularized via their editor – VSCode (one of the most popular dev tools) and supported by Google (Angular – the first big framework that recommended TypeScript) and Facebook (React has full TypeScript support).
- It is as flexible as JavaScript but provides advanced techniques know from more enterprise languages such as Java or C#.
- It is compatible with JavaScript, which means one can use any of the millions of JS packages in their TypeScript code.
Increased team flexibility – assemble your engineering team faster.
TypeScript can be used for all layers of your service (backend, frontend) and applied to all major runtime environments (mobile, desktop, browsers, servers). That versatility creates an opportunity to build a technically homogenous team. For instance, you don’t need to hire Python engineers for the web app’s backend and JavaScript engineers for the frontend – TypeScript engineers will handle both sides.
It’s not uncommon, especially in smaller teams, that someone from the team responsible for the backend needs to make some UI adjustments on the frontend or mobile app. With a shared tech-stack, such a task becomes much easier.
Good practices? Interesting technical discoveries? Perfect solutions? All those are often language-specific. Using TypeScript makes it possible to share knowledge and build a more collaborative environment.
Finally, it can help remove the communication gap between frontend and backend engineers and thus increase the overall team’s performance!
What's next?
If you are a developer
- To get started with TypeScript, we recommend the official “TypeScript for JavaScript programmers” guide and the “TypeScript in 50 Lessons” book.
- To continue learning TypeScript and JavaScript, check out our blog.
- For some immediate hands-on experience, why not check out (and even contribute to) OpenSource projects such as Prism (HTTP Mocking tool written in a functional TypeScript) or VSCode.
- To start a TypeScript engineer job, follow us on LinkedIn, Facebook, or Twitter to get notified about job opportunities, and join our team of top TypeScript engineers!
If you are a CTO/CEO looking for TypeScript engineers
- We provide team extension services for early-stage startups. Please check out our services page and read our Stoplight Case Study.
- Follow us on LinkedIn, Facebook, or Twitter to receive insights about the TypeScript ecosystem and more content similar to the one on this page.
- Want to chat about your team? Say hello via the chatbot or leave a note in the “Let’s get in touch” below. We’re also available on Messenger, Telegram, WhatsApp. If you prefer a more direct approach, feel free to reach out to our CEO, Chris Miaskowski, directly on his LinkedIn.